Vue Js Validate CVV Number:Vue.js is a JavaScript framework that allows developers to build user interfaces. To validate a CVV (Card Verification Value) number using Vue.js, you can use a combination of HTML input fields and Vue.js methods. First, you would bind the CVV input field to a Vue data property. Then, you can create a validation method that checks if the CVV input matches the required format, such as a three-digit number. This method can be triggered on form submission or input change events. If the CVV is valid, you can proceed with the desired action, such as submitting the form or displaying a success message.
How can I validate a CVV number using Vue js?
The provided code snippet demonstrates how to validate a CVV (Card Verification Value) number using Vue.js. It creates a Vue application with a data property cvv
and isValidCVV
flag initially set to true
. The input field is bound to the cvv
property using v-model
and the @input
event triggers the validateCVV
method.
Inside the validateCVV
method, any non-digit characters are removed from the cvv
value using a regular expression. The cleaned CVV is then checked for a valid length, which should be either 3 or 4 digits. The isValidCVV
flag is updated accordingly.
If the CVV entered is not valid, an error message is displayed using the v-if
directive.
Vue Js Validate CVV Number Example
<div id="app">
<label for="cvv">CVV Number:</label>
<input type="text" id="cvv" v-model="cvv" @input="validateCVV" />
<p v-if="!isValidCVV" class="error">Invalid CVV number.</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
cvv: '',
isValidCVV: true,
};
},
methods: {
validateCVV() {
// Remove any non-digit characters
const cleanedCVV = this.cvv.replace(/\D/g, '');
// CVV should be a 3 or 4 digit number
const isValidLength = cleanedCVV.length === 3 || cleanedCVV.length === 4;
// Update the validity flag
this.isValidCVV = isValidLength;
},
},
});
app.mount('#app');
</script>
Output of Vue Js Validate CVV Number
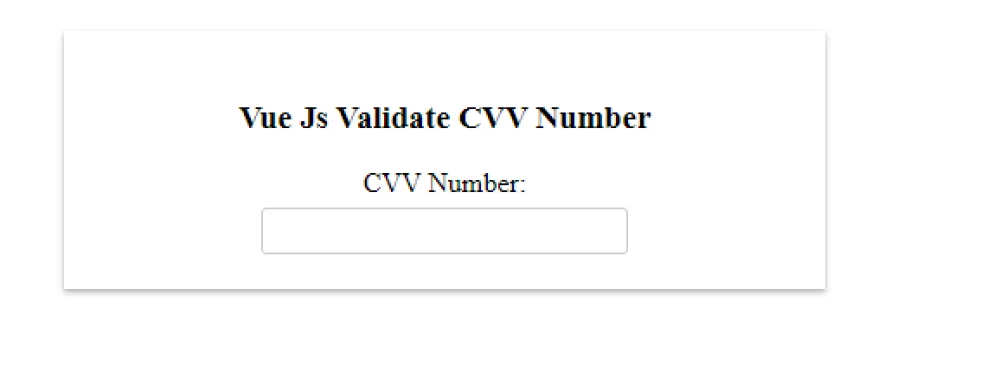